To comply with GDPR regulations and ensure lawful data processing, Doofinder provides a cookie consent validation feature. This article explains how to implement this functionality, its technical aspects, and how user experience is altered when cookies are declined.
By following the guidelines in this document, you can effectively integrate cookie consent functionality into your Doofinder-enabled application.
Altered Functionalities When Cookies Are Not Accepted
When cookie consent validation is enabled, users who do not accept cookies will experience reduced functionality. The affected features are:
Search:
- Latest searches and query retention are disabled.
- Users cannot scroll back to the last-clicked product after reopening the search layer.
- Altered statistics:
- Metrics such as
session_id
anduser_id
become unavailable. - Data on unique visitors becomes less accurate, and collisions may occur in cookieless tracking.
Recommendations:
- Browsing history functionality is disabled.
- Altered statistics:
- Session-based data, such as
user_id
, becomes unavailable. - Inaccuracies in visitor tracking may occur.
Quiz Maker:
- Altered statistics:
- Metrics such as
session_id
anduser_id
become unavailable. - Inaccuracies in visitor tracking may occur.
Enabling Cookie Consent Validation
The cookie consent validation can be activated in the store settings within the Doofinder Admin Panel. Once enabled, users who do not accept cookies will experience reduced functionality in the services provided by Doofinder. For further details, see the section Altered Functionalities above.
Activating this feature requires notifying Doofinder of the user's cookie acceptance to enable full functionality.
To activate this functionality, go to Configuration > Store Settings > in the first section you will find the “Cookie Consent for Full Product Functionality” toggle.
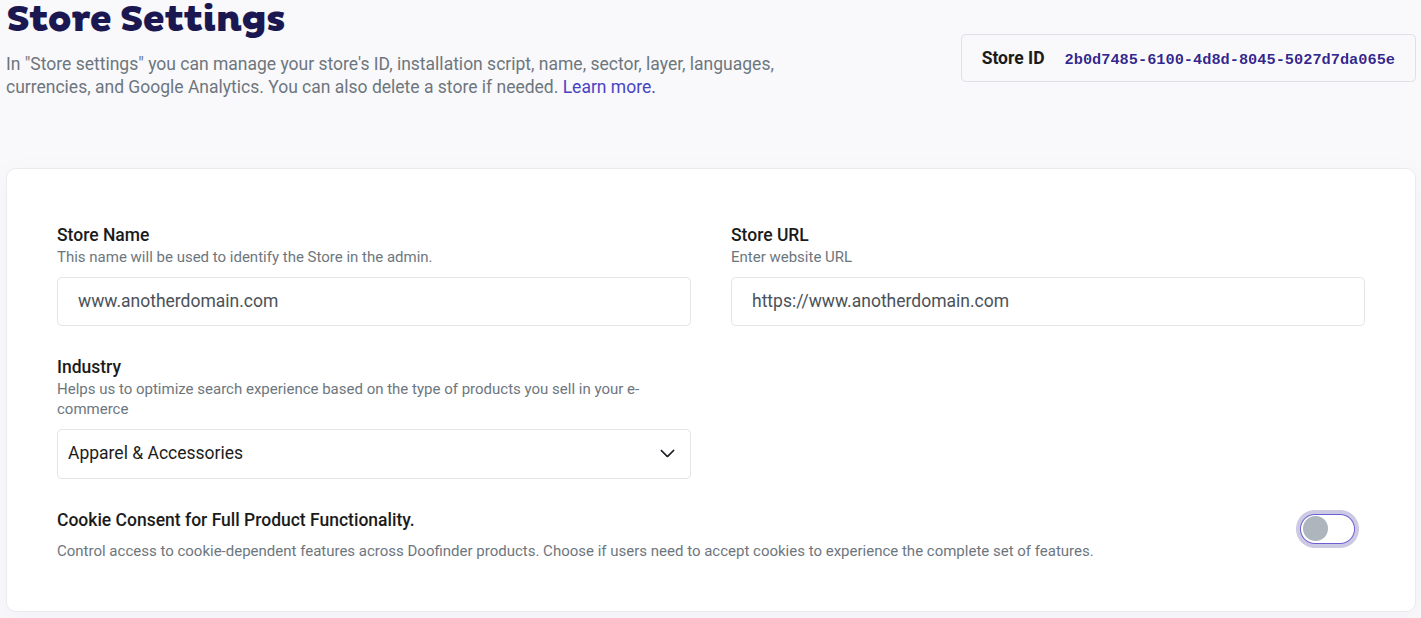
It is possible to override the default cookie consent settings. If needed, contact our support team to get help.
When cookie consent validation is enabled, it is essential to notify Doofinder when users accept cookies. This step ensures that all features of the products you are subscribed to are fully operational and available. By confirming user consent, Doofinder can provide its complete functionality, including analytics and tailored recommendations, without restrictions caused by cookie limitations.
This is achieved using the Doofinder.enableCookies()
function. Below is an example of how to implement this notification.
Implementation Examples
- Implementation in JavaScript: Cookie acceptance logic example.
<!-- Cookie Banner -->
<div id="cookieBanner">
<p>This site uses cookies to enhance your experience.</p>
<button id="acceptCookiesButton">Accept Cookies</button>
</div>
<script>
// Function to notify Doofinder and hide the banner
function acceptCookies() {
Doofinder.enableCookies(); // Notify Doofinder
document.getElementById("cookieBanner").style.display = "none"; // Hide banner
}
// Add event listener to the cookie acceptance button
document.getElementById("acceptCookiesButton").addEventListener("click", acceptCookies);
</script>
This structure ensures that when a user clicks the button to accept cookies, the Doofinder.enableCookies()
function is called to notify Doofinder.
- Implementation in Frameworks like React or Vue: React example.
import React from 'react';
function CookieBanner() {
const acceptCookies = () => {
Doofinder.enableCookies(); // Notify Doofinder
// Additional logic like updating component state
};
return (
<div>
<p>This site uses cookies to enhance your experience.</p>
<button onClick={acceptCookies}>Accept Cookies</button>
</div>
);
}
export default CookieBanner;
This approach can be adapted to any framework or platform to suit the application's architecture.
Handling Cookie Acceptance Before Script Load
In some cases, users may accept cookies before the Doofinder script is fully loaded. To handle this scenario, you can store a flag in LocalStorage as follows:
window.addEventListener("cookie-accepted", () => {
window.localStorage.setItem("df-cookies-allowed", "true");
});
This ensures that the user's consent is retained and synchronized once the Doofinder script becomes available.
Shopware V5 and V6
By default, in Shopware versions 5 and 6, the require_cookies_consent setting is enabled.
The Doofinder app automatically sends the user's cookie acceptance status. However, if a custom cookie manager is used, manual integration using the Doofinder.enableCookies()
function is required, as outlined in the examples above.
Conclusion
Implementing cookie consent validation ensures compliance with GDPR and similar regulations while offering users the choice to accept or decline cookies. Although declining cookies may result in reduced functionality, the implementation of the feature is straightforward and flexible, accommodating diverse platforms and frameworks.